Understanding Next.js Image: The Ultimate Guide for Developers
Discover the power of Next.js Image component for image optimization. Learn how it automatically optimizes images, facilitates lazy loading, and serves modern formats, enhancing website performance and responsiveness.
Matija
If you're familiar with modern web development frameworks, you've likely come across Next.js, an open-source React framework developed by Vercel. One notable feature of Next.js is the Image component, a powerful utility for streamlining image optimization in web applications. In this comprehensive guide, we'll delve deep into the inner workings of Next.js Image, its use cases, misconceptions, limitations, and advantages.
How Next.js Image Works Under the Hood
The primary objective of the Next.js Image component is to facilitate image optimization. It automatically optimizes images as they are requested, implementing inlining images, lazy loading, resizing, and serving modern image formats. These actions can lead to significant performance improvements for your web application.
When a user requests an image, the Image component sends a request to the Next.js server. The server then retrieves the original image, performs necessary optimizations, and returns the optimized image to the browser. The server caches the optimized image to serve future requests faster.
Here's a basic example of how to use the Next.js Image component:
import Image from 'next/image';
<Image
src="/path/to/image.jpg" // Path to your image
height={500} // Image height
width={500} // Image width
alt="Description of image" // Alt text for accessibility
/>
Handling Internal (Local) and External Images
You can use the Next.js Image component to serve both internal and external images. For internal images, you only need to specify the path to the image file as in the above example. However, for external images (those not located in the public directory), you need to specify the domains of these images in the 'next.config.js' file:
module.exports = {
images: {
domains: ['example.com'], // Add the domain here
},
};
After specifying the domains, you can use the external image in the same way as the local ones:
<Image
src="https://example.com/path/to/image.jpg" // Use the full URL
height={500}
width={500}
alt="Description of image"
/>
.jpg)
Customizing deviceSizes and imageSizes
The 'next.config.js' file also allows you to customize 'deviceSizes' and 'imageSizes'. 'deviceSizes' is used to define the sizes of viewport devices, while 'imageSizes' is used to specify sizes of images. By default, Next.js uses five values for 'deviceSizes' and 'imageSizes'. Modifying these arrays can affect how images are served and rendered, optimizing your application for specific devices or visual layouts.
Please note that changing these values affects the image generation process, and unnecessary alterations could lead to inefficiencies. Therefore, only adjust these values if you understand the implications and have a specific need.
Limitations and Misconceptions
While the Next.js Image component offers substantial benefits, it does have certain limitations:
Image cropping: The component can resize images, but it does not support cropping. If you need to display only a part of an image, you'll have to manually crop it before serving.
Mandatory width and height: The Image component requires the 'width' and 'height' attributes to be explicitly defined. This requirement is part of how Next.js optimizes image delivery by ensuring the correct size of the image is sent based on the consuming device's screen resolution. You can't use the Image component without specifying these attributes. If you try to do so, it'll lead to an error. However, using the 'layout' property set to 'fill' or 'responsive' allows the image to resize based on its container.
Server-Side Rendering (SSR) and Static Site Generation (SSG): As of now, the Image component only supports client-side rendering. Images aren't pre-rendered with SSR or SSG, which might cause some delay in image loading.
Restricted to Next.js environment: As a part of the Next.js framework, the Image component can't be used in projects that don't use Next.js.
Configuration for External Images: As mentioned earlier, to work with external images, you must add their domains to the 'next.config.js' file.
In terms of misconceptions:
The Next.js Image component is not a drop-in replacement for the HTML 'img' tag. Although it provides additional functionalities, you can still use the 'img' tag where image optimization is not critical or when you need more control over the image, such as applying specific CSS styles or interactions that the Image component does not support.
Advantages of Next.js Image
Despite its limitations, the Next.js Image component offers several compelling advantages:
Automatic Optimization: The component optimizes images on-demand, thereby improving website performance and reducing bandwidth usage.
Responsive Images: By setting the 'sizes' property, images can adapt to the screen size and resolution, enhancing your website's responsiveness.
Lazy Loading: Images are only loaded when they come into the viewport, which improves initial page load time.
Modern Formats: The component automatically serves images in modern formats like WebP when the browser supports it, further reducing image sizes.
Conclusion
In conclusion, the Next.js Image component is a robust tool for optimizing website images. Although it has a few limitations, its advantages significantly outweigh them, especially for performance-focused applications. Armed with this guide, you're now ready to leverage the power of image optimization in your Next.js applications.
We hope you found this article insightful. Please feel free to share your thoughts and experiences with the Next.js Image component in the comments section below. Happy coding!
Articles You Might Like
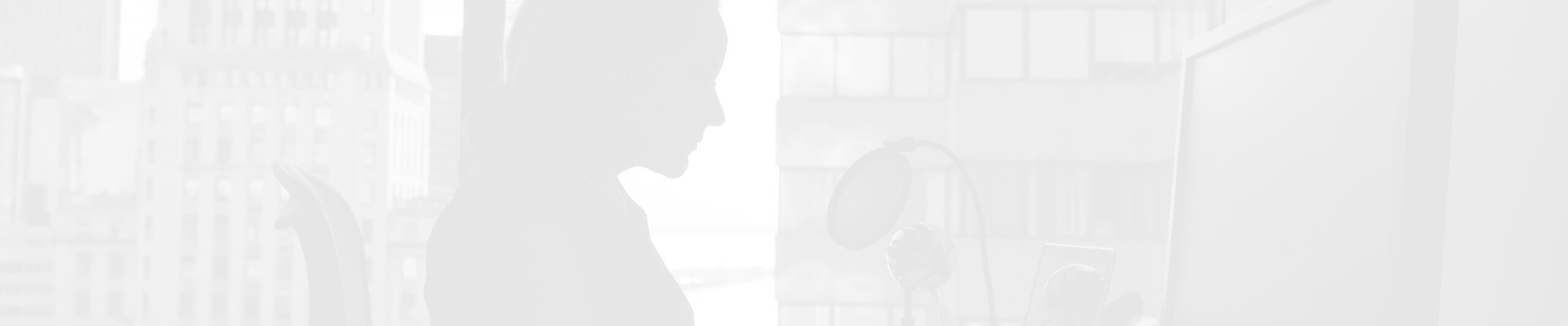