Clean SCSS
As developers, it is crucial to prioritize the creation of clean, maintainable, and well-documented code. By adhering to established methodologies, principles, conventions, and architecture, we can ensure that our CSS code is clean, simple, and easy to maintain. In this blog post, we will explore some essential practices for writing clean SCSS code.
Matej
Utilizing Best Practices
To achieve clean code, we are using the following best practices:
DRY (Don't Repeat Yourself): The DRY principle in software development emphasizes the reduction of code repetition. By avoiding duplications, we can improve maintainability and reduce the chances of introducing bugs.
7-1 Sass Architecture: The 7-1 Sass Architecture is a widely used approach that organizes CSS files into seven folders and one main file. This structure helps to effectively sort and structure the codebase, making it easier to navigate and maintain.
BEM (Block Element Modifier): BEM is a naming convention and methodology that promotes clear, self-documenting CSS code. By defining blocks, elements, and modifiers, we can create meaningful class names that improve code readability and maintainability.
Careful Namespacing: Careful namespacing involves choosing appropriate and descriptive names for CSS classes. By using prefixes such as 'u-' for utility classes, 't-' for theme-related classes, and 'is-' or 'has-' for temporary or state-based classes, we can enhance code clarity and avoid naming conflicts.
"Outside In" Ordering of CSS Properties: The "Outside In" approach suggests ordering CSS properties based on their impact on the element from the outside to the inside. Following this order, we prioritize layout properties, box model properties, visual properties, typography properties, and miscellaneous properties. This ordering improves code readability and makes it easier to locate specific properties.
.jpg)
Applying the Best Practices
To demonstrate the application of these best practices, let's consider an example component. Keep in mind that this example is not designed or responsive and may not adhere to best coding practices.
Example Component:
HTML file:
<div id=”accordion” class="accordion">
<h2 id=”accordion-title” class="accordion-headline">
<button class="accordion-button”>
<span>What is Flowbite?</span>
<span class=" icon”>X</span>
</button>
</h2>
<div id="accordion-body" class="body-accordion">
<div class="body-accordion-wrapper">
<p class="">Content part 1 …</p>
<p class="-">Content part 2 …
<a href="https://example" class="link">Link Example</a>
</p>
</div>
</div>
</div>
SCSS file:
.accordion {
background-color: black;
color: white;
border: 1px solid green;
margin: 5rem auto;
max-width: 50%;
}
.accordion-title {
color: red;
pointer: cursor;
margin-right: 1rem;
padding: 2rem;
}
.accordion-button {
font-size: 1.5rem;
justify-content: space-between;
align-content: center;
display: flex;
.icon {
font-size: 1.25rem;
height: 1.5rem;
width: 1.5rem;
}
}
.accordion-body {
overflow: hidden;
max-height: 0;
.is-open-accordion & {
max-height: 100%;
}
}
.accordion-content {
padding: 2rem;
}
Improvement Steps
Replace IDs with classes: Instead of using IDs for element selection, it is better to use classes with a 'js-' prefix to indicate their purpose for JavaScript functionality. This approach promotes separation of concerns and improves code maintainability.
Use BEM structure and naming convention: Apply BEM to define blocks and elements, taking advantage of SCSS nesting for improved readability and easy design adjustments. Ensure that the SCSS elements are ordered according to their importance and appearance.
Extract reusable components: Identify frequently used components and extract them into separate SCSS files. For example, a button component can be defined and styled independently, making it reusable throughout the project.
Improve namespacing: Add appropriate prefixes to classes based on their nature. Use 'c-' for components, 'u-' for utility classes, and follow BEM's naming convention for blocks, elements, and modifiers.
Apply the "Outside In" approach: Order the CSS properties within each class based on the "Outside In" principle, starting with layout properties, followed by box model properties, visual properties, typography properties, and miscellaneous properties. This ordering enhances readability and makes it easier to locate specific properties.
Result of Applying Best Practices
After applying these best practices, the code will look more professional and maintainable:
HTML file:
<div class="accordion">
<h2 class="accordion__headline" data-accordion-heading>
<button class="accordion__button">
<span>What is Flowbite?</span>
<span class="accordion__icon">X</span>
</button>
</h2>
<div class="accordion__body" data-accordion-body>
<div class="accordion__content">
<p>Content part 1 …</p>
<p>Content part 2 …
<a href="https://example" class="accordion__link">Link Example</a>
</p>
</div>
</div>
</div>
SCSS file:
.accordion {
&__headline {
margin-right: 1rem;
padding: 2rem;
color: red;
cursor: pointer;
}
&__button {
font-size: 1.5rem;
justify-content: space-between;
align-content: center;
display: flex;
&__icon {
font-size: 1.25rem;
height: 1.5rem;
width: 1.5rem;
}
}
&__body {
overflow: hidden;
max-height: 0;
&.is-open-accordion {
max-height: 100%;
}
}
&__content {
padding: 2rem;
}
}
Conclusion
Writing clean CSS code brings numerous benefits to developers and clients alike. By adopting best practices and focusing on maintainability and efficiency, developers can work more effectively and deliver high-quality results. Prioritizing clean code reduces the likelihood of bugs, improves code readability, and promotes code reuse. Ultimately, these practices lead to satisfied clients and a positive development experience.
Articles You Might Like
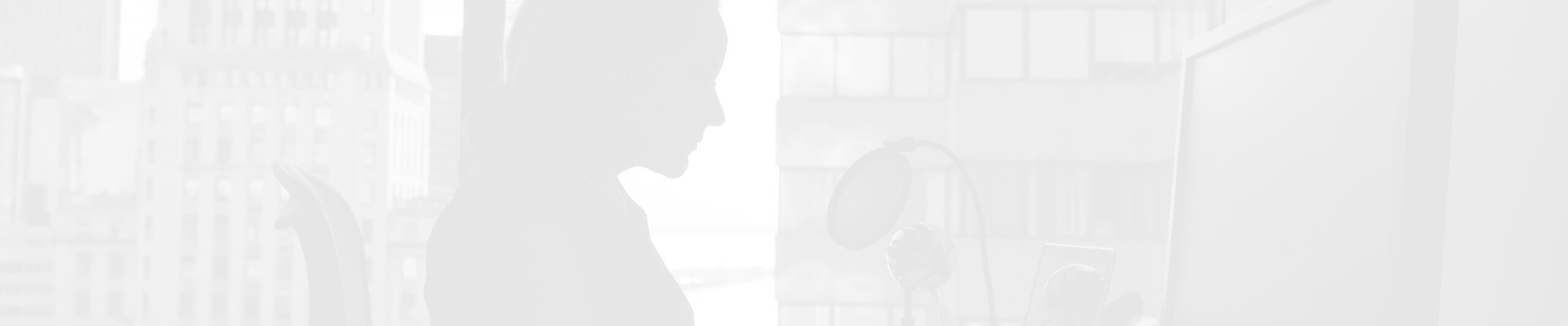